The Notification Service Extension modifies the notification content by adding actions, push polls and allows you to take advantage of iOS 10+ notification features.
Add new target to your project (
File->New->Target
) and create Notification Service Extension, and name itPushbotsNSExtension
, Press cancel on the "ActivatePushbotsNSExtension
scheme" prompt:
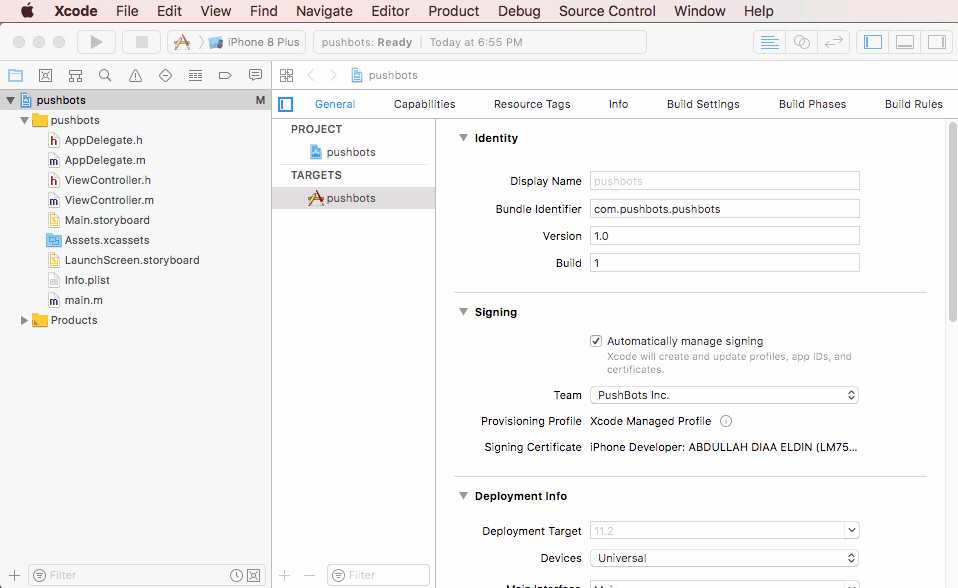
Make sure deployment info > Target of the Notification Extension Service is set to 10 or higher under General tab.
2. Open NotificationService.m
or NotificationService.swift
under PushbotsNSExtension Group
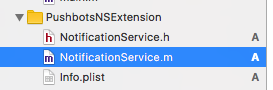
and copy the code below:
Objective-C NotificationService.m
// NotificationService.m
#import <Pushbots/Pushbots.h>
#import "NotificationService.h"
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;
@property (nonatomic, strong) UNNotificationRequest *notificationRequest;
@end
@implementation NotificationService
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler {
self.notificationRequest = request;
self.contentHandler = contentHandler;
self.bestAttemptContent = [request.content mutableCopy];
[Pushbots didReceiveNotificationExtensionRequest:self.notificationRequest withContent:self.bestAttemptContent];
self.contentHandler(self.bestAttemptContent);
}
- (void)serviceExtensionTimeWillExpire {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
[Pushbots serviceExtensionTimeWillExpireRequest:self.notificationRequest withContent:self.bestAttemptContent];
self.contentHandler(self.bestAttemptContent);
}
@end
Swift NotificationService.swift
// NotificationService.swift
import UserNotifications
import Pushbots
class NotificationService: UNNotificationServiceExtension {
var contentHandler: ((UNNotificationContent) -> Void)?
var bestAttemptContent: UNMutableNotificationContent?
var notificationRequest: UNNotificationRequest!
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
self.contentHandler = contentHandler
self.notificationRequest = request;
bestAttemptContent = (request.content.mutableCopy() as? UNMutableNotificationContent)
if let bestAttemptContent = bestAttemptContent {
Pushbots.didReceiveNotificationExtensionRequest(self.notificationRequest, with: self.bestAttemptContent)
contentHandler(bestAttemptContent)
}
}
override func serviceExtensionTimeWillExpire() {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
if let contentHandler = contentHandler, let bestAttemptContent = bestAttemptContent {
Pushbots.serviceExtensionTimeWillExpireRequest(self.notificationRequest, with: self.bestAttemptContent)
contentHandler(bestAttemptContent)
}
}
}
Ignore any error till you finish step 2 to import PushBots SDK:
https://www.pushbots.help/install-pushbots-in-your-app-or-website/ios/the-pushbots-part-integrating-pushbots-sdk-into-your-ios-project
----
Flutter SDK additional config:
1. open Podfile, add:
target 'PushbotsNSExtension' do
Use_frameworks!
Use_modular_headers!
flutter_install_all_ios_pods File.dirname(File.realpath(__FILE__))
end
2. choose 'PushbotsNSExtension' target and go to Build Settings then disable Bitcode

3. Finally switch to ios folder in terminal and type pod install