Required before Integrating the SDK
PushBots account.
Firebase server key and sender ID (How?)
A device or emulator with google play services installed.
Android studio 2.3+
Step 1: Import the PushBots library into your project:
Open build.gradle (Module: app) file:
Add PushBots dependency along with Play services:
implementation 'com.android.support:appcompat-v7:27.1.0'
implementation 'com.android.support:support-v4:27.1.1'
implementation 'com.google.firebase:firebase-messaging:20.2.4'
implementation 'com.pushbots:pushbots-lib:3.3.3@aar'
Please make sure you're using latest Android Support library 27.1.0+
2. Add to defaultConfig section, then replace PUSHBOTS_APP_ID and GOOGLE_SENDER_ID:
manifestPlaceholders = [manifestApplicationId : "${applicationId}",
pushbots_app_id : "PUSHBOTS_APP_ID",
pushbots_loglevel : "DEBUG",
google_sender_id : "GOOGLE_SNEDER_ID"]
3. Sync Project with updated gradle file:
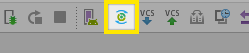
Step 2: Add required code:
Add to MyApplication class to application tag:
android:name=".MyApplication"
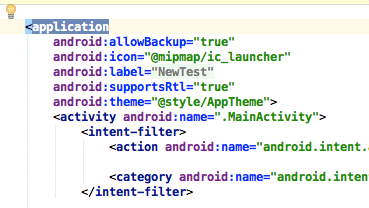
Click on warning icon and press alt + Enter
then select Create class 'MyApplication'
:
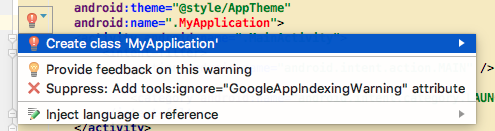
Initialize PushBots instance in it, copy the following just below [package ….;] line, and replace FCM_APPID, WEB_API_KEY, PROJECT_ID, SENDER_ID and PUSHBOTS_API_ID:
You can find all these credentials in this article:
https://www.pushbots.help/en/articles/498201-the-google-part-firebase-credentials
Java:
import android.app.Application;
import com.pushbots.push.Pushbots;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Initialize Pushbots Library
new Pushbots.Builder(this)
.setFcmAppId("FCM_APPID")
.setLogLevel(Pushbots.LOG_LEVEL.DEBUG)
.setWebApiKey("WEB_API_KEY")
.setPushbotsAppId("PUSHBOTS_APP_ID")
.setProjectId("PROJECT_ID")
.setSenderId("SENDER_ID")
.build();
}
}
Kotlin:
import android.app.Application
import com.pushbots.push.Pushbots
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
// Initialize Pushbots Library
Pushbots.Builder(this)
.setFcmAppId("FCM_APPID")
.setLogLevel(Pushbots.LOG_LEVEL.DEBUG)
.setWebApiKey("WEB_API_KEY")
.setPushbotsAppId("PUSHBOTS_APP_ID")
.setProjectId("PROJECT_ID")
.setSenderId("SENDER_ID")
.build()
}
}
That's pretty much it. Run your application now, if all goes well, your device should be registered and ready to receive push notifications!
Building Customer profiles:
PushBots lets you convert devices into humans, by tying devices to users to see who they are, what they have done in your app, and to give you a compelling way to search, find them and communicate with them using it.
//Register custom fields after user registered on PushBots
Pushbots.sharedInstance().idsCallback(new Pushbots.idHandler() {
@Override
public void userIDs(String userId, String registrationId) {
if (registrationId != null && userId != null){
Log.d("PB3", "Registration ID:" + registrationId + " | userId:" + userId);
//Customer profile
Pushbots.sharedInstance().setFirstName("John");
Pushbots.sharedInstance().setLastName("Smith");
Pushbots.sharedInstance().setName("John smith");
Pushbots.sharedInstance().setGender("M");
Pushbots.sharedInstance().setEmail("a@aa.cc");
Pushbots.sharedInstance().setPhone("+20");
}
}
});