Step 1: Import PushBots Plugin:
Add the PushBots plugin to config.xml:
<gap:plugin name="pushbots-cordova-plugin" spec="1.6.14" source="npm" />
<preference name="android-minSdkVersion" value="19" />
<preference name="android-build-tool" value="gradle" />
<preference name="phonegap-version" value="cli-9.0.0" />
Please make sure you're using phonegap sdk 8.1.0+ to support android 8/Oreo.
Step 2: Add Required code:
Add this line to your js/index.js to intialize Pushbots in onDeviceReady section:
window.plugins.PushbotsPlugin.initialize("PUSHBOTS_APPLICATION_ID", {"android":{"sender_id":"GOOGLE_SENDER_ID"}});
// Only with First time registration
window.plugins.PushbotsPlugin.on("registered", function(token){
console.log("Registration Id:" + token);
});
//Get user registrationId/token and userId on PushBots, with evey launch of the app even launching with notification
window.plugins.PushbotsPlugin.on("user:ids", function(data){
console.log("user:ids" + JSON.stringify(data));
});
You should replace PUSHBOTS_APPLICATION_ID with the application ID found in Keys tab in app settings, and GOOGLE_SENDER_ID with the senderID generated using this guide.
Methods to use it:
window.plugins.PushbotsPlugin.setAlias("Test");
window.plugins.PushbotsPlugin.removeAlias();
//Add or remove Single tag
window.plugins.PushbotsPlugin.tag("tag1");
window.plugins.PushbotsPlugin.untag("tag1");
//Add or remove array of tags
window.plugins.PushbotsPlugin.setTags(["tag1"]);
window.plugins.PushbotsPlugin.removeTags(["tag1"]);
window.plugins.PushbotsPlugin.debug(true);
//unsubscribe user from receiving notifications
window.plugins.PushbotsPlugin.toggleNotifications(false);
//iOS only
//Reset Badge
window.plugins.PushbotsPlugin.resetBadge();
//Set badge
window.plugins.PushbotsPlugin.setBadge(10);
//Increment badge count
window.plugins.PushbotsPlugin.incrementBadgeCountBy(1);
//Decrement badge count
window.plugins.PushbotsPlugin.decrementBadgeCountBy(10);
To handle Notification events:
// Should be called once app receive the notification [foreground/background]
window.plugins.PushbotsPlugin.on("notification:received", function(data){
console.log("received:" + JSON.stringify(data));
//iOS: [foreground/background]
console.log("notification received from:" + data.cordova_source);
//Silent notifications Only [iOS only]
//Send CompletionHandler signal with PushBots notification Id
window.plugins.PushbotsPlugin.done(data.pb_n_id);
});
window.plugins.PushbotsPlugin.on("notification:clicked", function(data){
// var userToken = data.token;
// var userId = data.userId;
console.log("clicked:" + JSON.stringify(data));
});
Step 3: Build and test your app.
Update your code and rebuild.
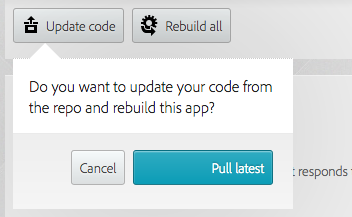